Use data to grow your business
Get trusted team of Data Engineers & Scientists that will increase your company’s revenue
Services
Looking for data masters? Look no further. Our senior data consultants will grow your business with personalized solutions in Machine Learning, Generative AI, and Data Science & Analytics.
DS STREAM solutions
Apache Airflow Managed Service
We simplify pipeline automation and scaling, allowing your team to focus on insights and strategy rather than maintenance. Leave the heavy lifting to us—your data deserves better.
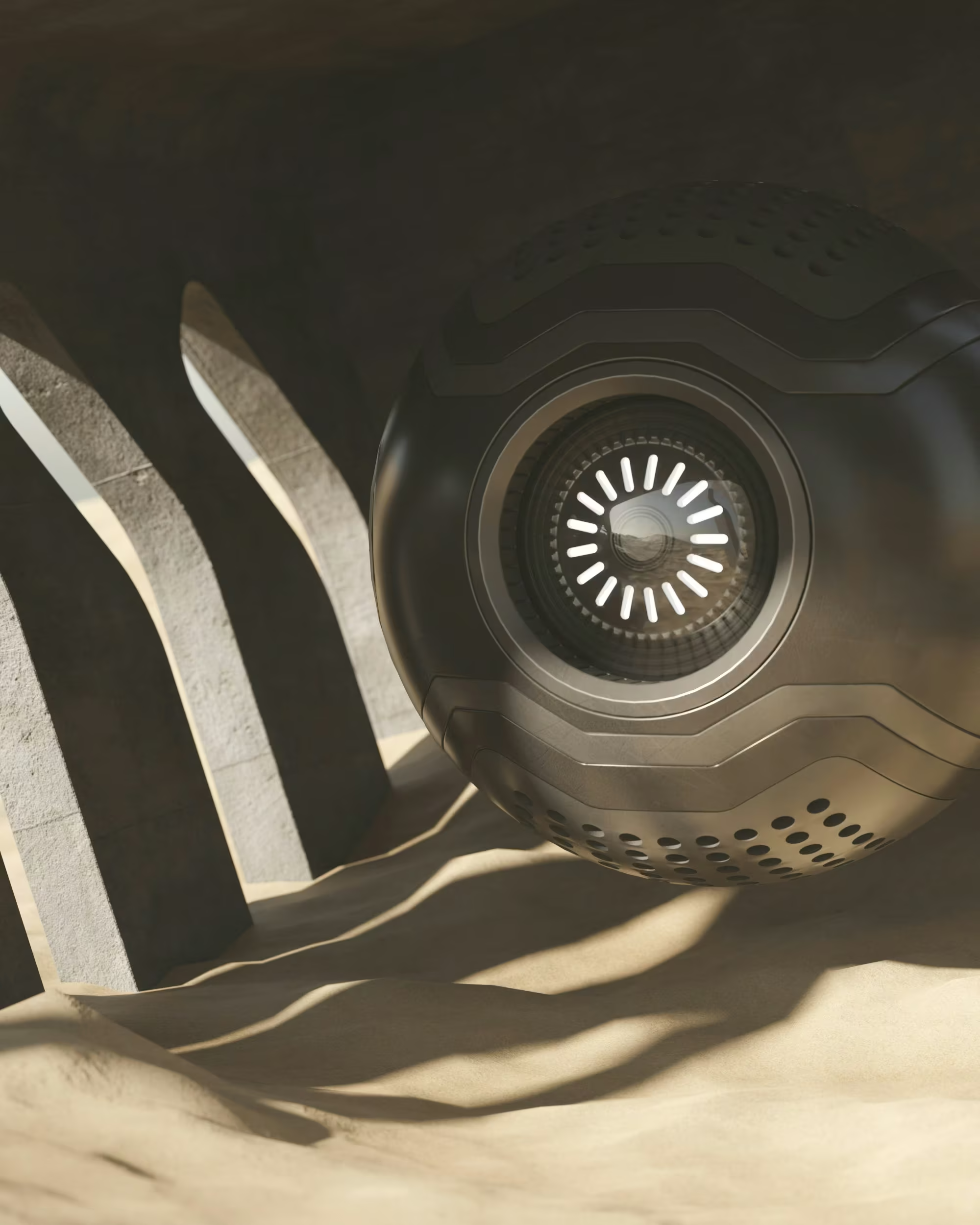
Machine Learning & MLOps
Our mission is to integrate Machine Learning technologies with DevOps practices for a seamless transition from development to deployment. We automate Machine Learning workflows to boost efficiency and predictability, offering tailored MLOps solutions on your infrastructure or in the cloud.
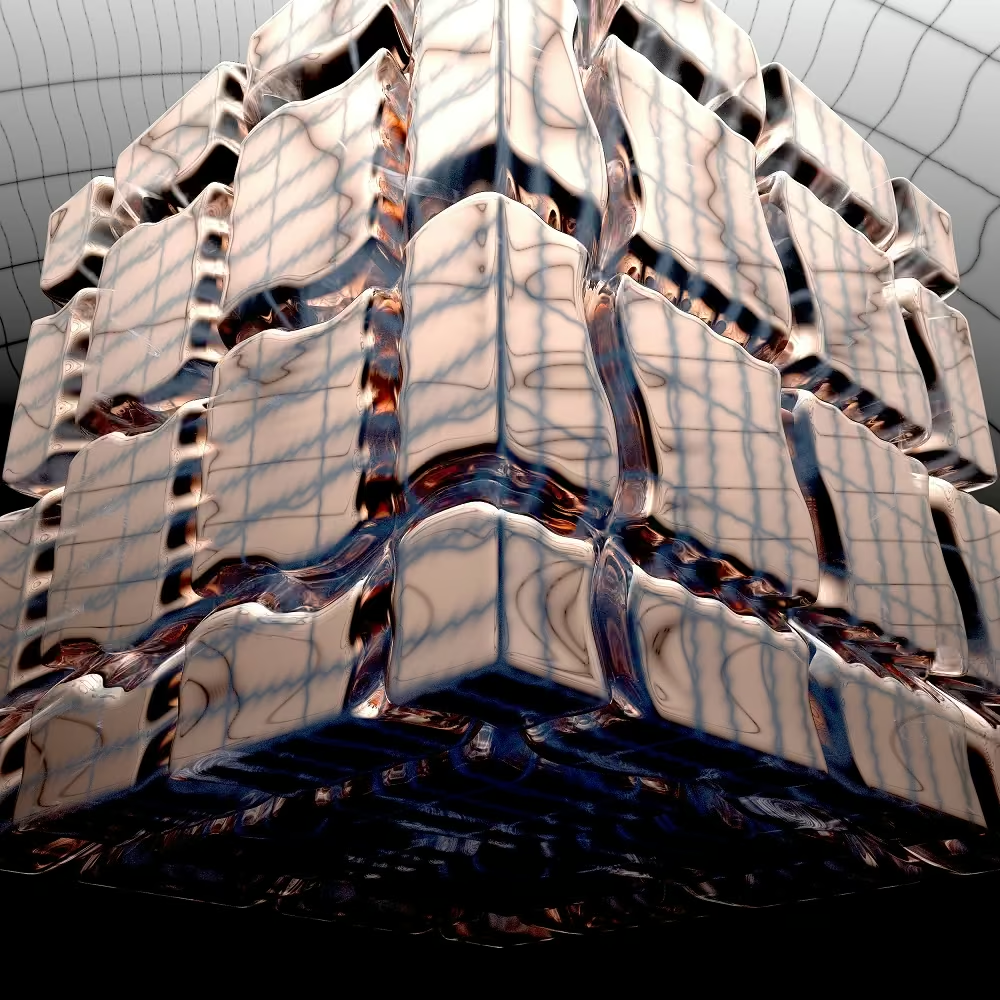
Generative AI
Discover modern AI technologies that bring innovative solutions. Leverage the expertise of our data, AI, and Generative AI experts to unlock the full potential of AI technology. We will provide you with the custom AI tools and support in AI-driven business decisions.

Data Science & Analytics
With our advanced Data Science and Analytics services, you will gain full insights into your company's data, turning data into real profit.
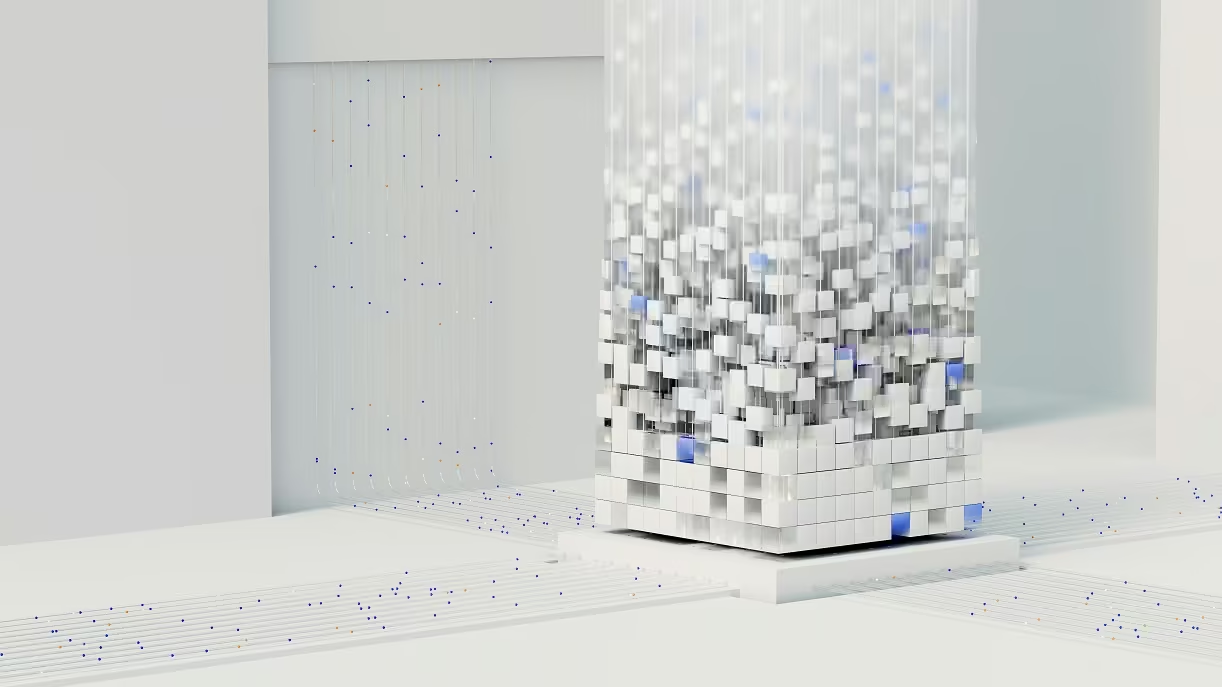
Data Engineering
Our Data Engineering experts transform disparate data into the valuable knowledge you need to make better-informed business decisions.
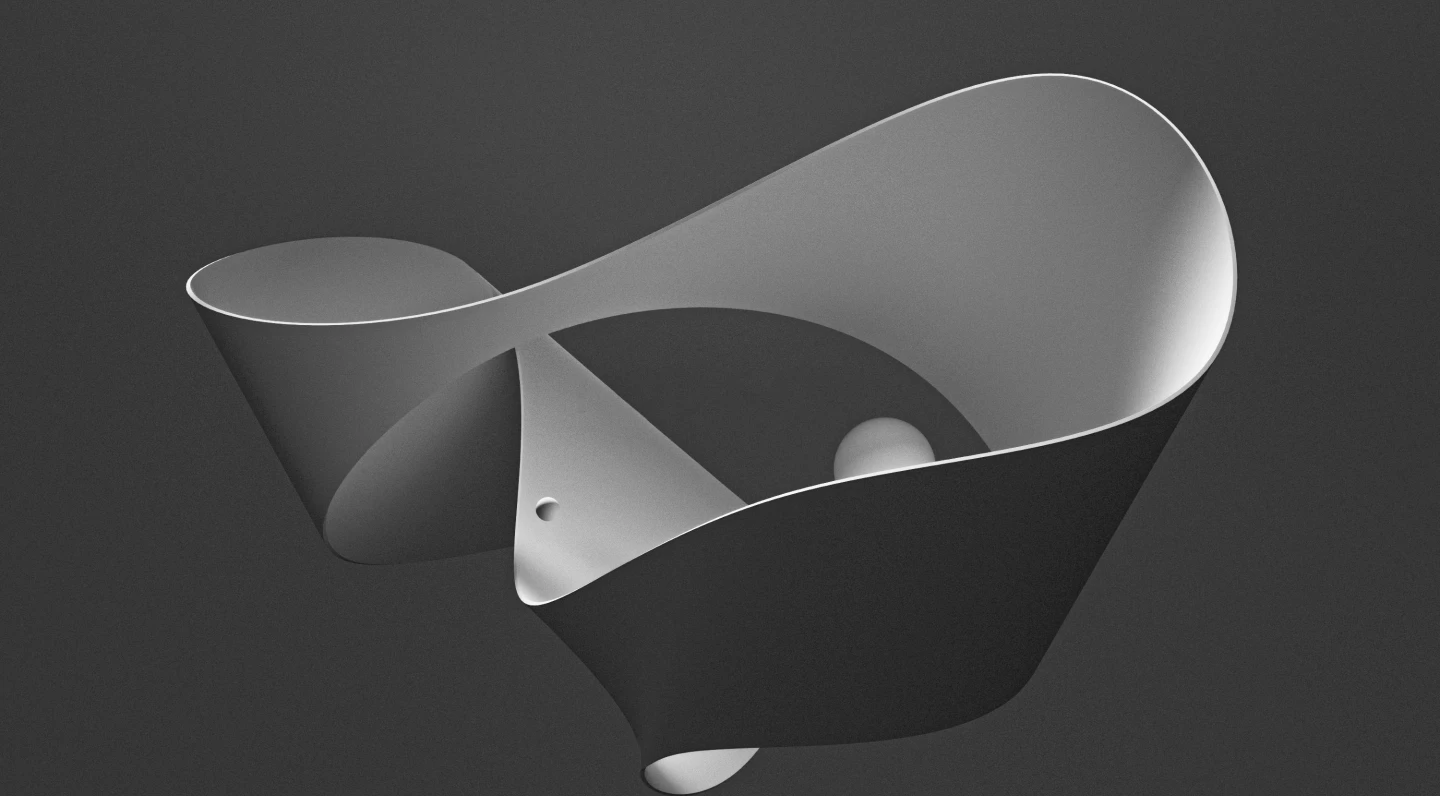
Cloud Solutions
Streamline your business processes by moving them to the cloud with our Cloud Solutions, gaining access to fast and reliable solutions anywhere and anytime.

Software Engineering
We build software tailored to your needs with expertise in Software Engineering. Our engineers explore your business, understand what the product needs to grow, and use the best technology to thrive it.

Apache Airflow Managed Services
We simplify pipeline automation and scaling, allowing your team to focus on insights and strategy rather than maintenance. Leave the heavy lifting to us—your data deserves better.
Industries
Work with data consultants who tackle business challenges every day. We help companies in sectors such as FMCG, Retail, E-commerce, Healthcare, and Telecommunications boost their revenue and profitability. Our data services is designed especially to drive financial growth.

FMCG
Providing Data Science and Analytics solutions for the Fast-Moving Consumer Goods industry to optimize supply chains and increase sales.

Retail
Leveraging Analytics and Machine Learning to enhance customer experiences in the Retail sector.

E-commerce
Implementing Data Science and Machine Learning to drive E-commerce growth and efficiency.
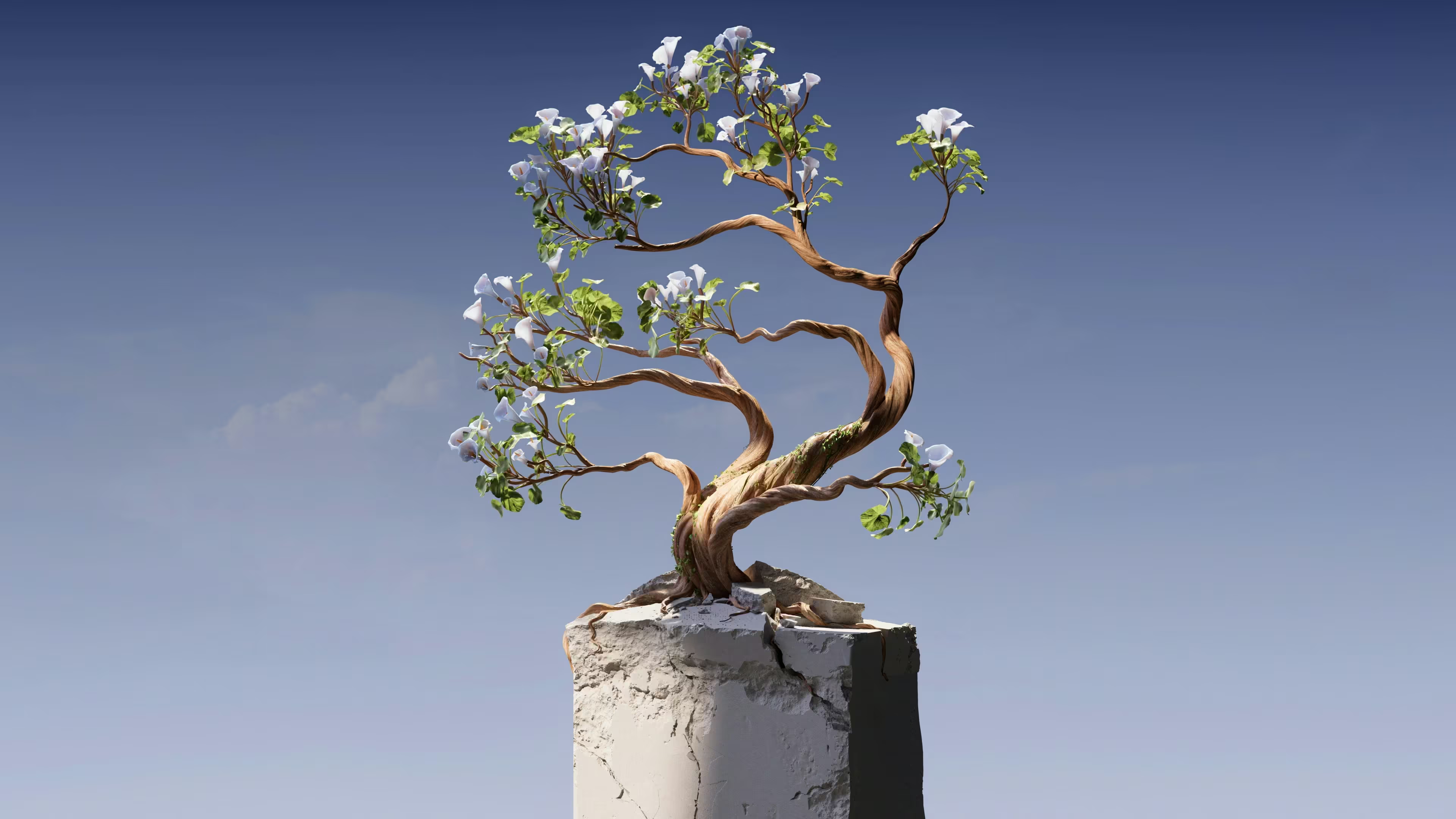
Healthcare
Applying Advanced Analytics and Cloud Solutions to improve outcomes and operational efficiency in Healthcare.
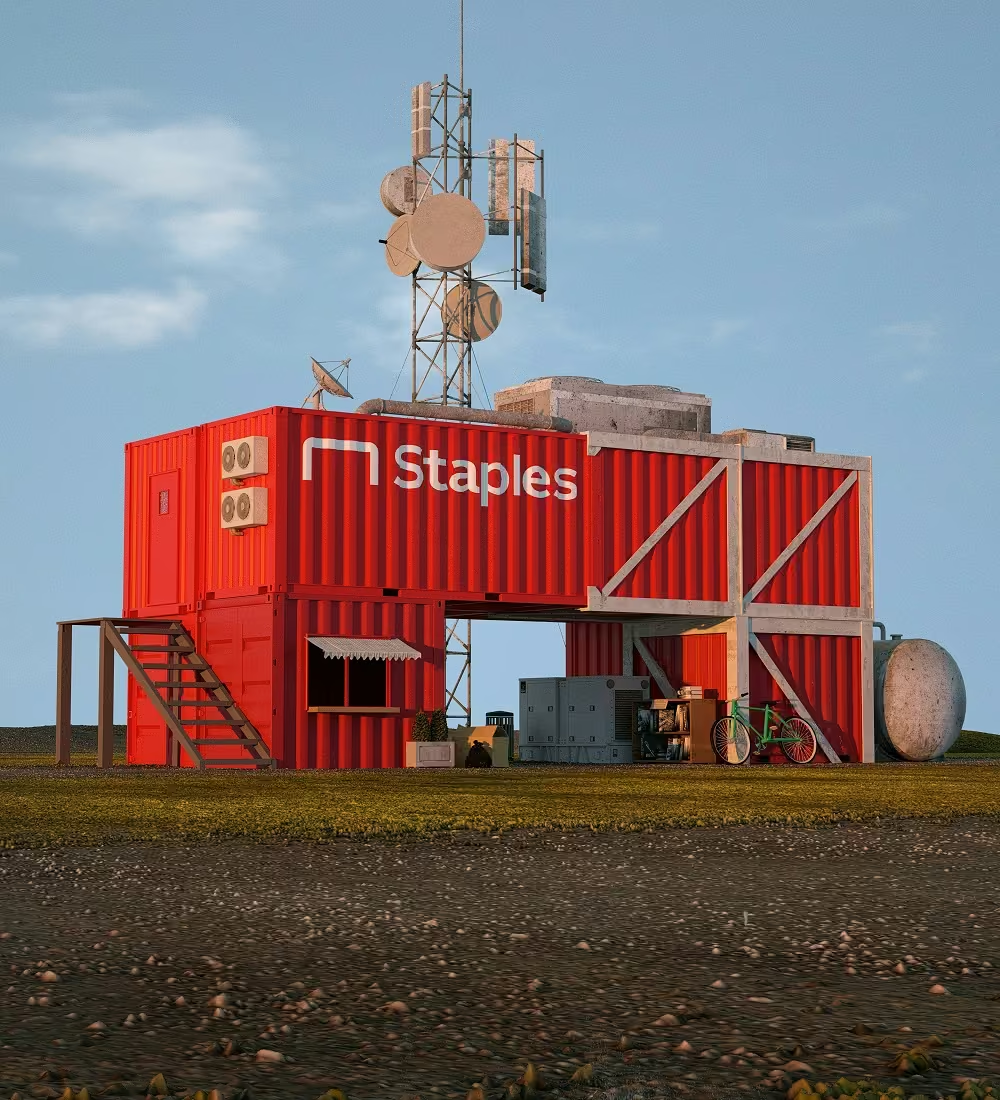
Telecommunications
Utilizing MLOps and Generative AI to innovate and streamline Telecommunications services.

Global Trade Intelligence
Unlock real-time visibility, predictive power and operational agility across the world’s supply chains

FMCG
Providing Data Science and Analytics solutions for the Fast-Moving Consumer Goods industry to optimize supply chains and increase sales.
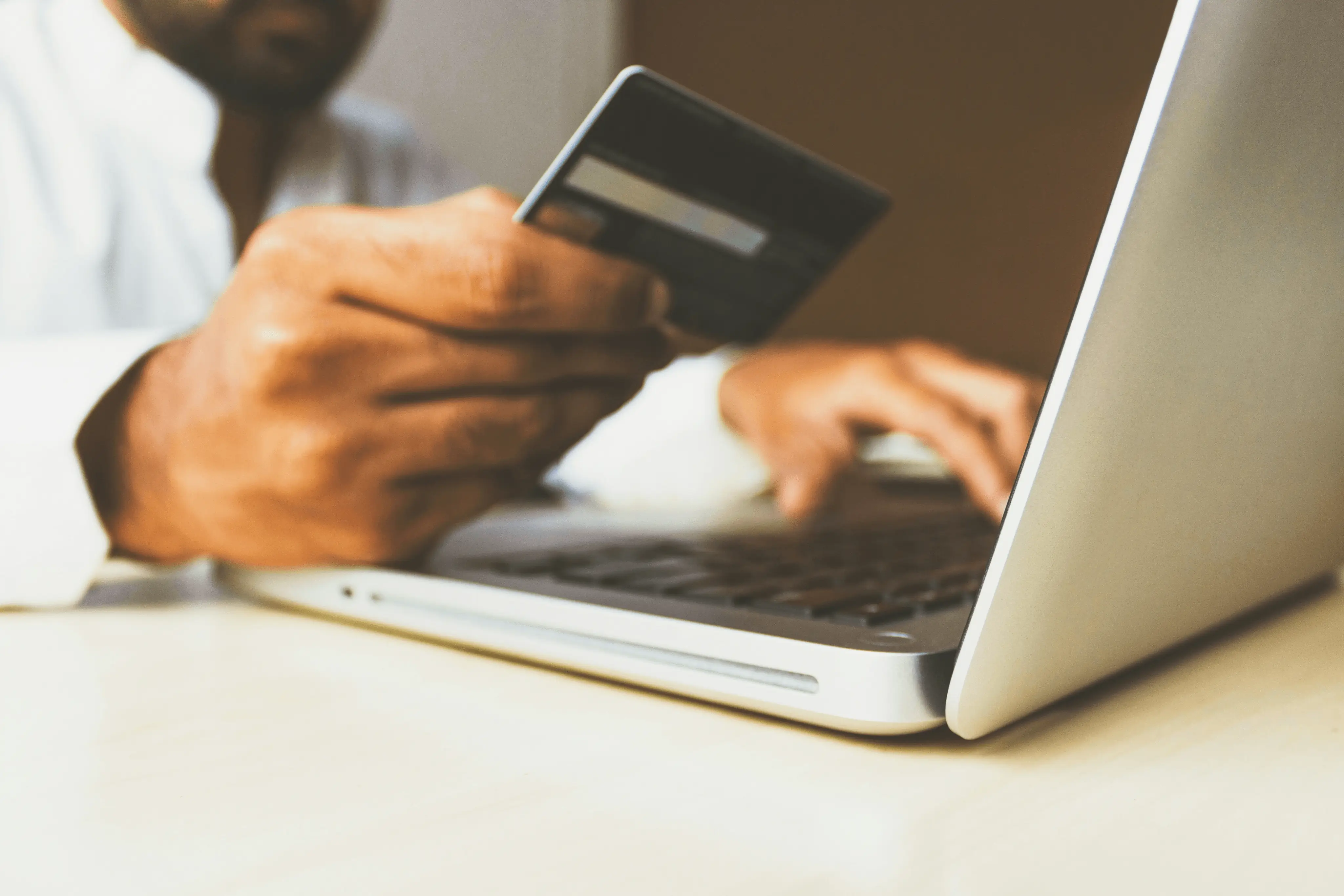
E-commerce
Implementing Generative AI and Cloud Solutions to drive E-commerce growth and efficiency.
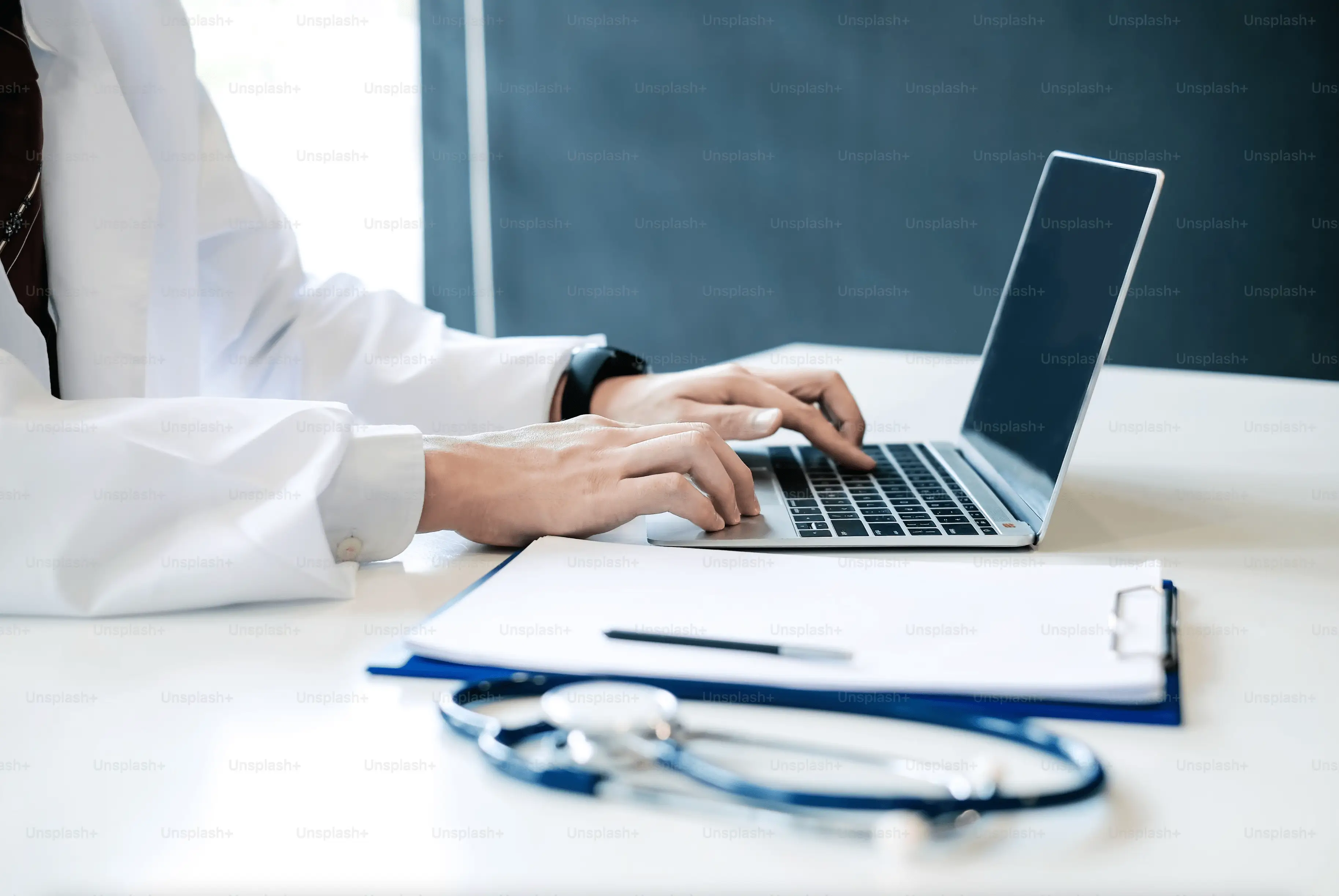
Healthcare
Applying advanced Analytics and AI to improve patient outcomes and operational efficiency in Healthcare.
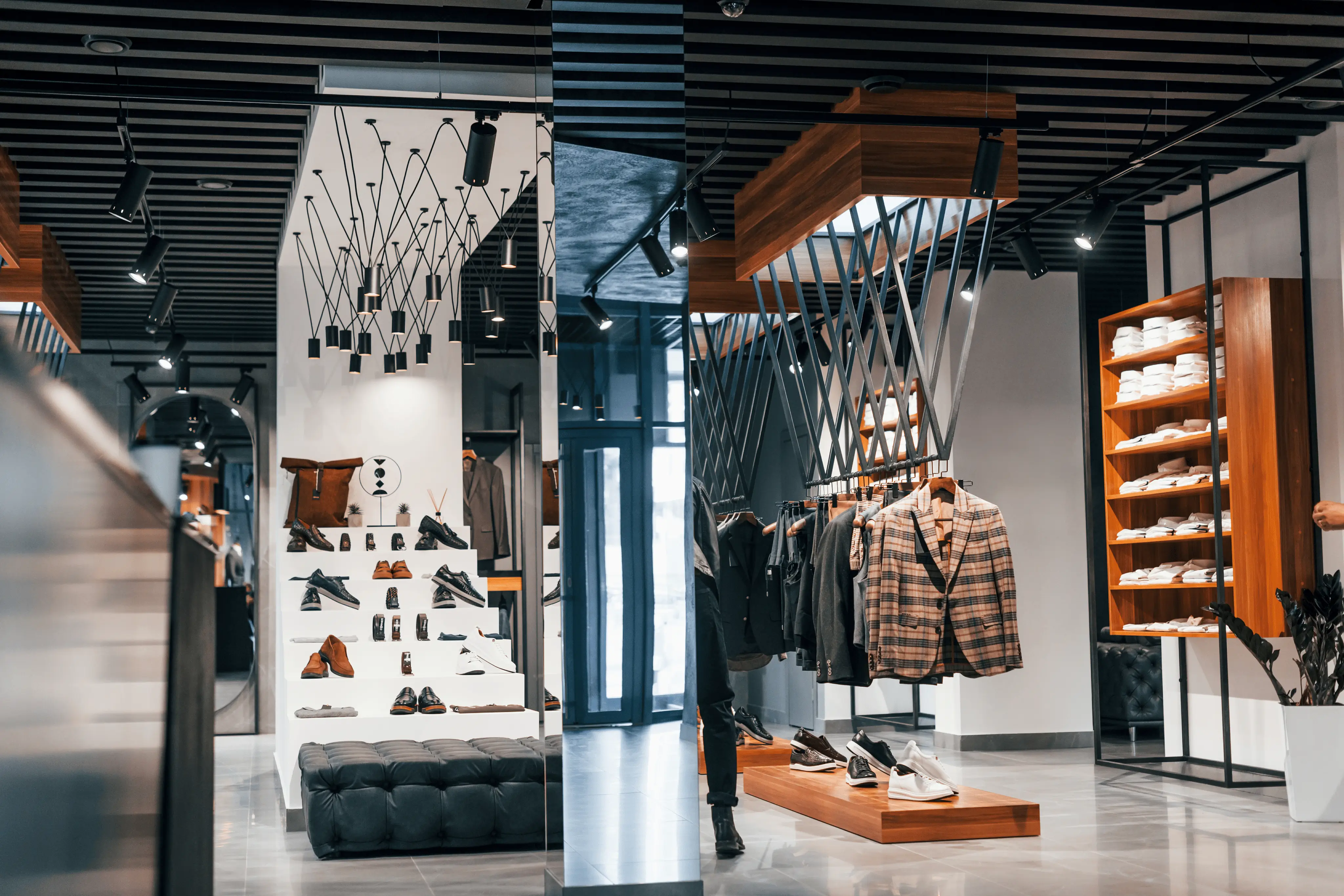
Retail
Leveraging Machine Learning and Data Engineering to enhance customer experiences in the Retail sector.
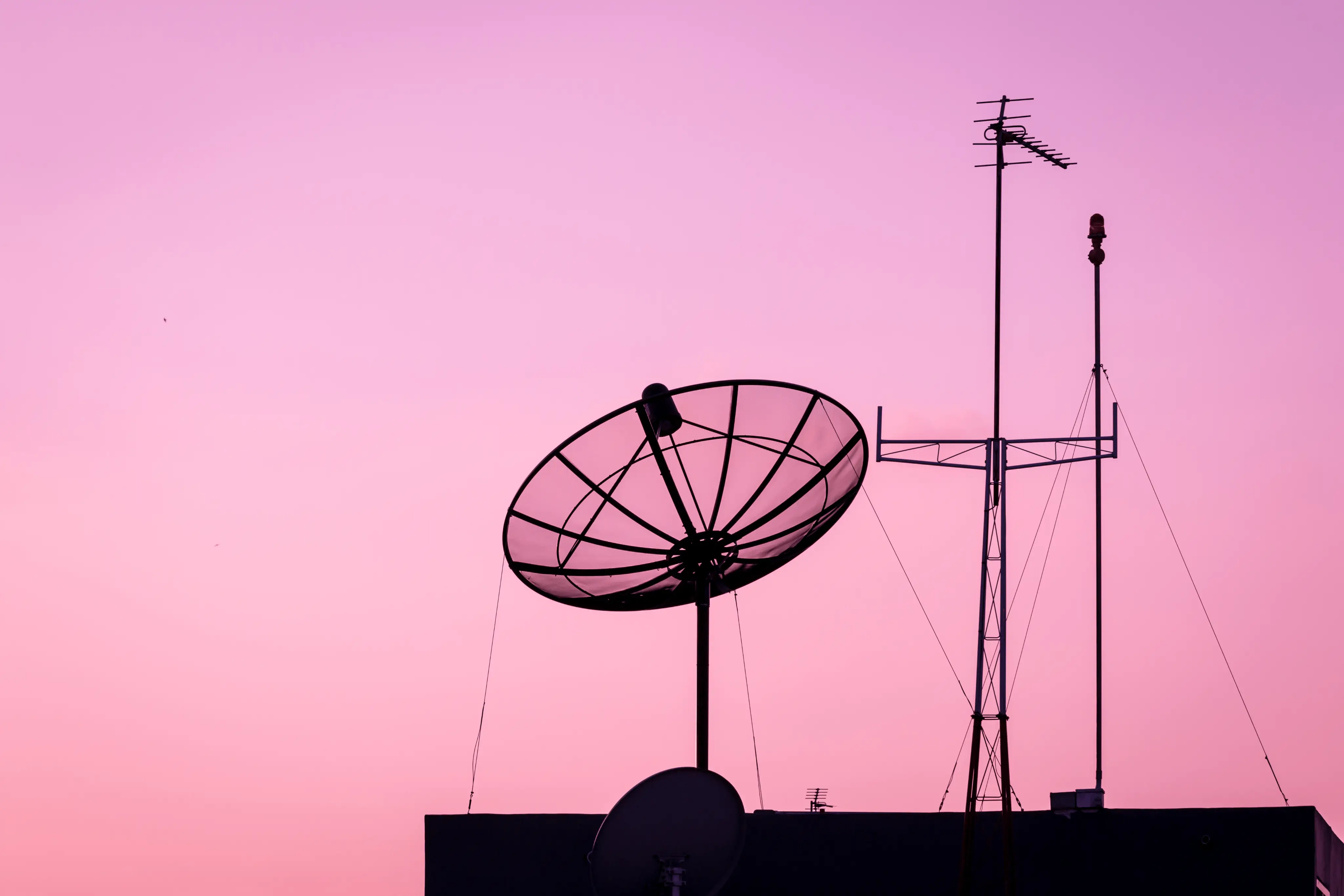
Telecommunications
Utilizing Data Engineering and MLOps to innovate and streamline Telecommunications services.
Experience
With many years of cross-industry experience at a senior level in advanced analytics, Data Science, Machine Learning Operations, and Generative AI solutions, we deliver excellence. Take a look at some case studies and discover why our clients value our teams’ work.
What our clients say
Gen Yang
Data Science Manager, Kpler
Anonymous
CEO, Sports Analytics Company
Maciej Mościcki
CEO, Macmos Stream
Adam Murray
Head of Product Development, Sportside
Gen Yang
Data Science Manager, Kpler
Anonymous
CEO, Sports Analytics Company
Maciej Mościcki
CEO, Macmos Stream
Adam Murray
Head of Product Development, Sportside
Selected Clients
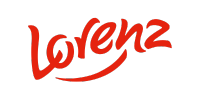
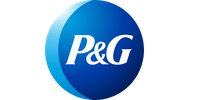
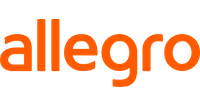
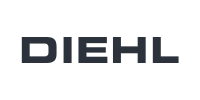
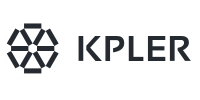
DS STREAM in numbers
Professionals on board
Years on market
Project delivered
Technologies mastered
Certifications archieved
Industries we perform
Drop us a line and check how Data Engineering, Machine Learning, and AI experts can boost your business.
Talk to expert – It’s free

Why choose us?
DS STREAM is an experienced consulting company focused on analytics data management, AI and innovative solutions for global brands from all over the world. Every day, our senior data experts use the potential of AI and data analytics to provide complex solutions and innovation in various industries, remaining agility and technology agnostic approach.
Wide Industry Experience
We deliver advanced analytics in FMCG, retail, e-commerce, healthcare, telco, finance, logistics and more.
Senior teams
Our engineers have over 10 years of experience in Machine Learning, Data Science, and GenAI.
Advanced Analytics Expertise
Every day we provide tailored and complex data analytics for global corporations.
Tech agnostic approach
Our data consultants follow a technology agnostic approach. They conduct workshops and provide tools your business actually needs.

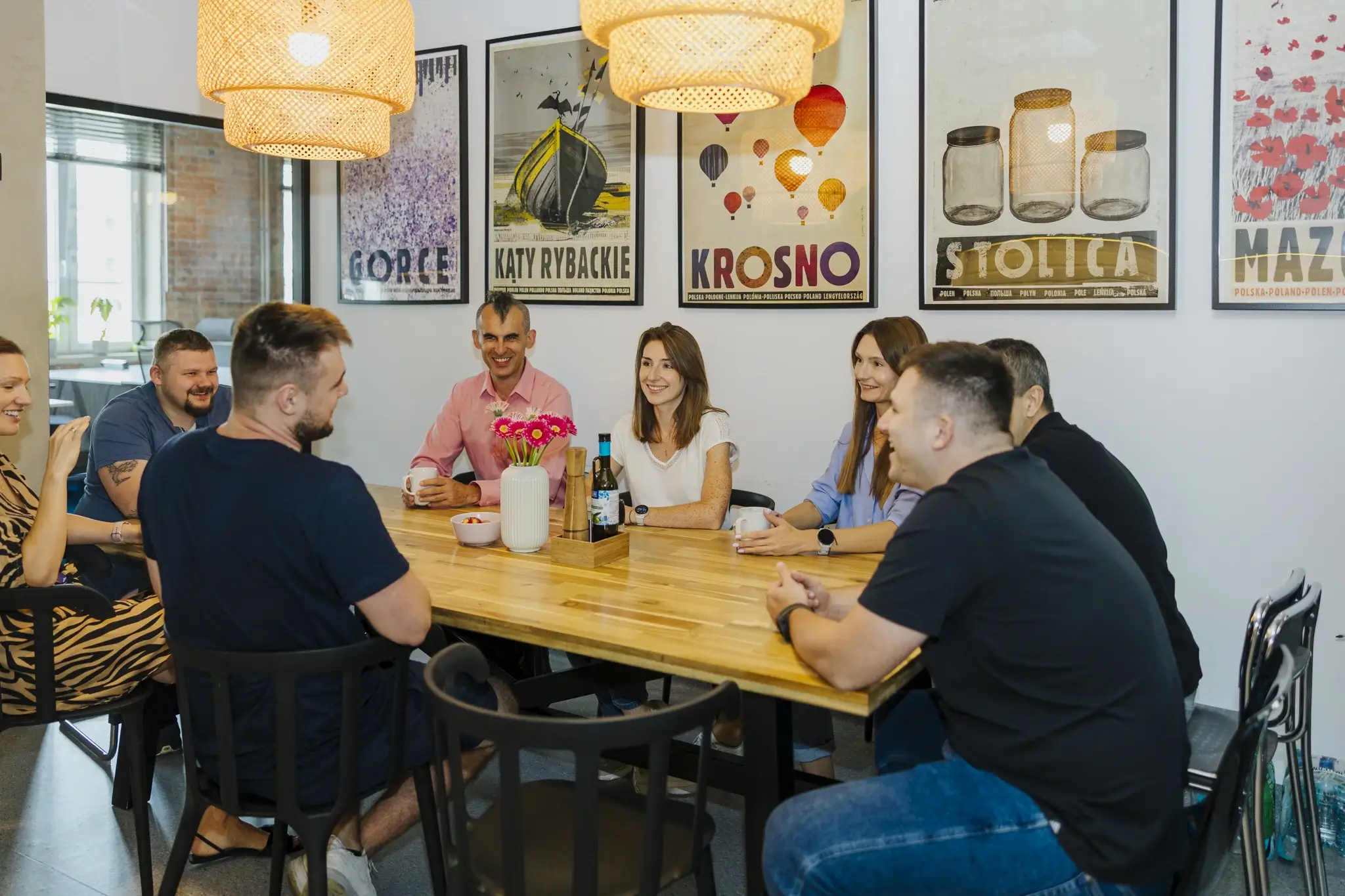
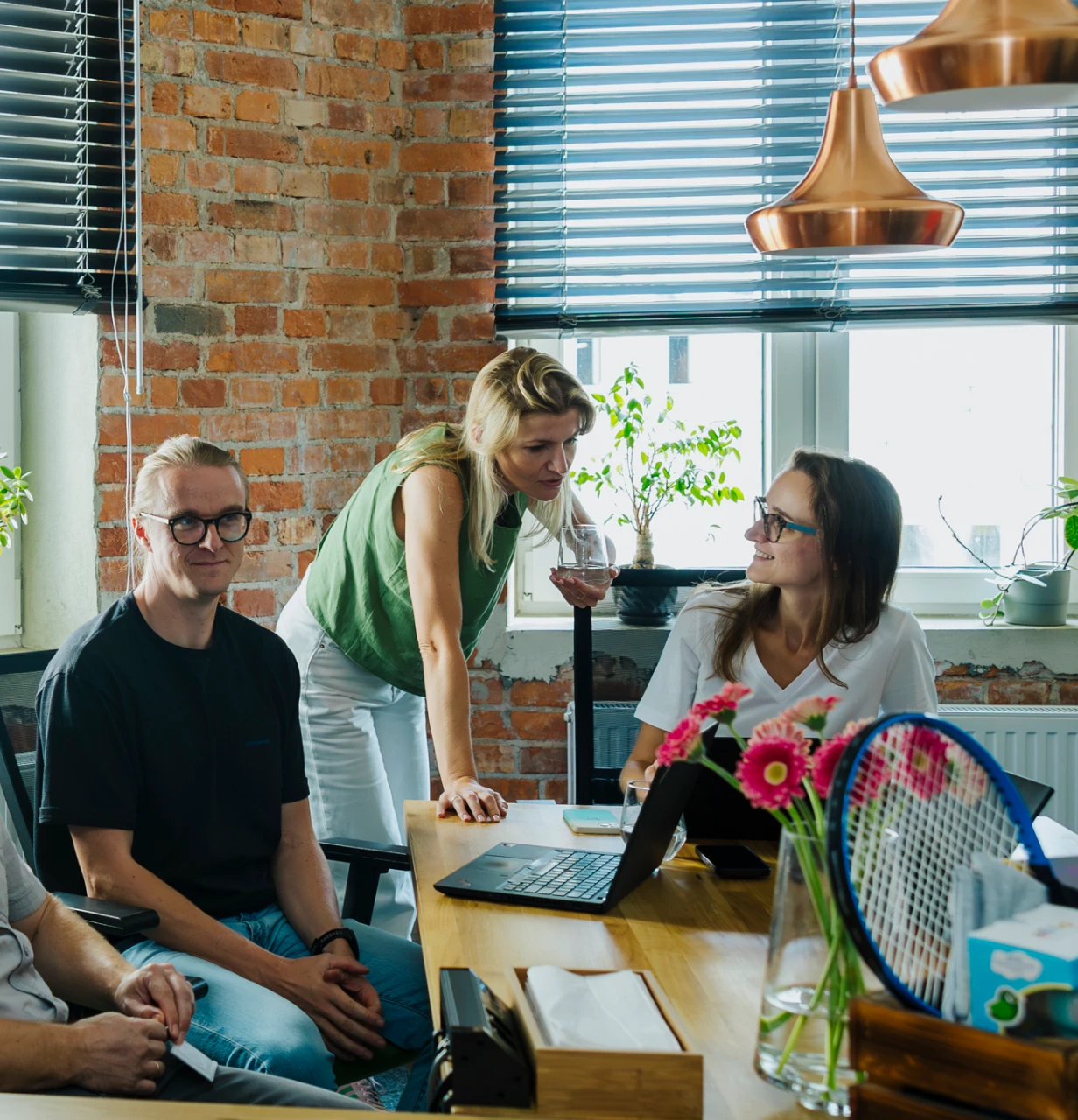


News & Blog
Discover our latest news and data-related blog posts
Let’s talk and work together
We’ll get back to you within 4 hours on working days
(Mon – Fri, 9am – 5pm CET).

Service Delivery Partner